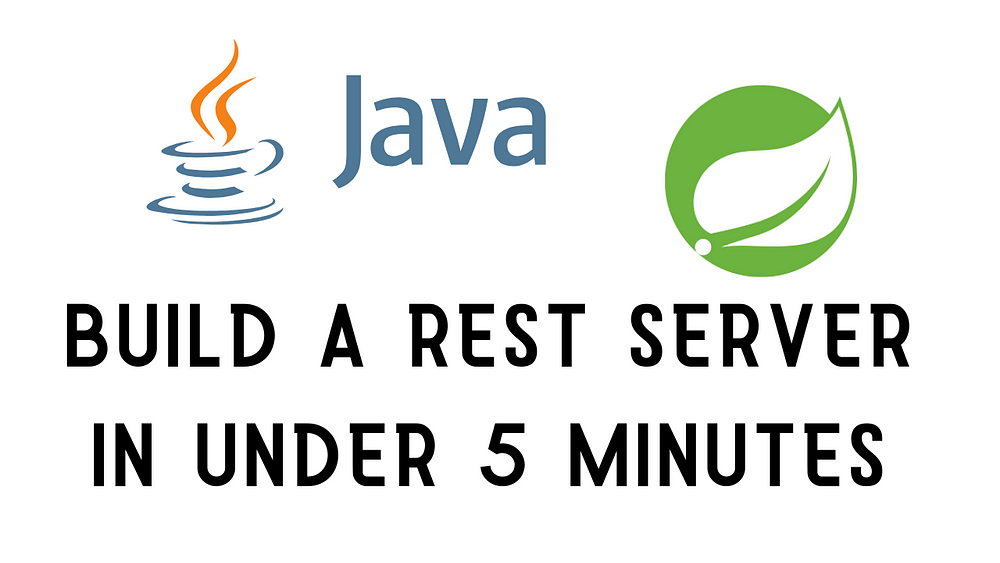
Today, we will be creating a rest server using Spring Boot in under 5 minutes.
To get started head on to
start.spring.io
In our starter project we will need the following dependencies:
- Spring Web
- Spring Data JPA
- Rest Repositories
- H2 Database (In memory database)
It should look like:

Now, we import the project into our IDE, in my case I am using IntelliJ .
Your imported project will look something like this:
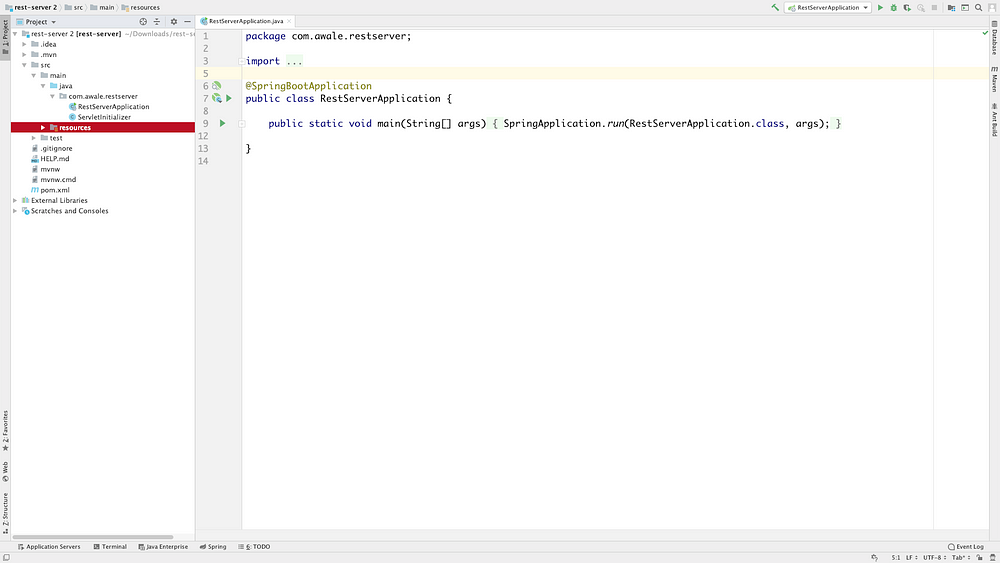
Lets create a Entity object which is basically a Table in your Database. In a file called main/java/com/awale/restserver/entity/Player.java
import javax.persistence.*;
@Entity
@Table(name="players")
public class Player {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
private String position;
private String team;
// Include Getters and setters
}
Now we need something that will handle the database connections, sql statements and everything related to persistence. This will be done by our PlayerRepository which will extend a JPARepository which does the actual magic.
In file main/repository/PlayerRepository.java
import org.springframework.data.jpa.repository.JpaRepository;@Repository
public interface PlayerRepository extends JpaRepository<Player, Long> {
}
This is all the code that is required to expose our Player entity to our rest server. We just have to add in a few configurations according to the database we are using. Since we are using an H2 database, we configure it as follows.
In main/resources/application.properties
spring.h2.console.enabled=true // console acts like a db manager
spring.h2.console.path=/h2
spring.datasource.url=jdbc:h2:mem:item
Since we are using a H2 database which is an in memory database we will add some db statements that insert data to test our rest server.
In main/resources/data.sql
INSERT Into Players (first_name,last_name,position,team) values ('Ram','Daniel', 'Striker', 'AFC');
INSERT Into Players (first_name,last_name,position,team) values ('Rob','Bott', 'Striker', 'AFC');
INSERT Into Players (first_name,last_name,position,team) values ('Trevor','Carry', 'Striker', 'AFC');
INSERT Into Players (first_name,last_name,position,team) values ('Trad','Daniel', 'Striker', 'AFC');
And this is it. You can run the application and test your rest server at localhost:8080/players
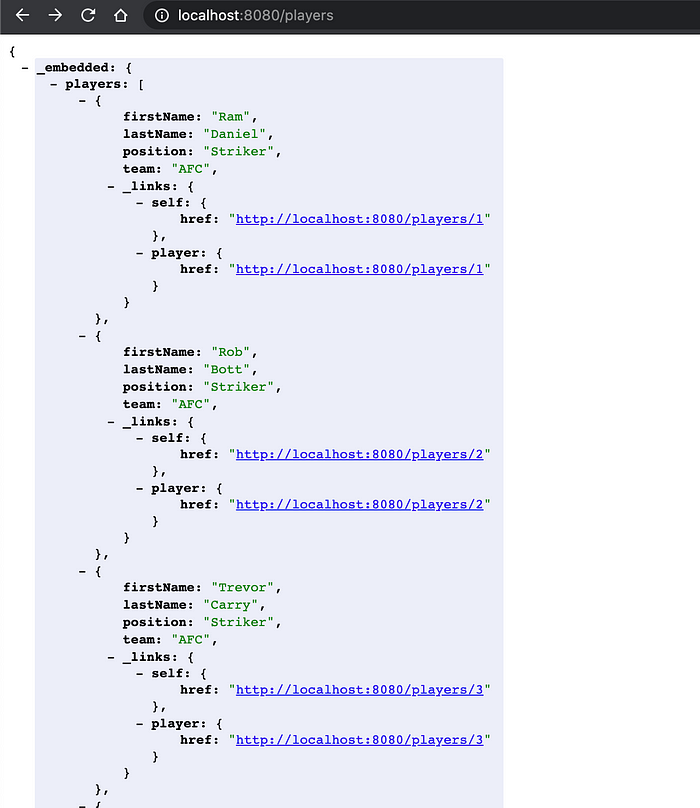
Thank you for reading. Please hit the like button if you found this useful.
The video guide is present below.